Templating With Maven Archetype
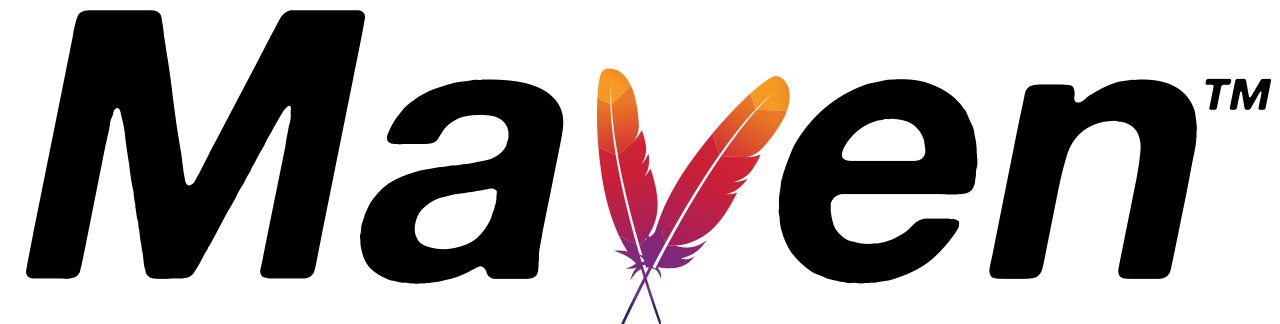
How to create your own template for a Maven project using Maven archetypes.
Maven archetypes helps you creating templates for you projects, so you can start them easily just like the well known Spring Initialzr.
Assumptions
It’s necessary to have Maven installed, and for more information about how to install Maven, please see this official documentation
A knowledge in the basics of Maven
Maven Archetype structure
Let’s discuss more about the structure of a Maven archetype. Maven archetype has:
A file that is the archetype descriptor, that goes on one of these two paths:
- src/main/resources/META-INF/maven/archetype.metadata.xml
- src/main/resources/META-INF/maven/archetype.xml
This descriptor defines the required properties of your archetype, as well as files that will be included.
Prototype files, that goes on:
- src/main/resources/archetype-resources
The propotype files are basically all of your project’s files, that you serve as template. These files also include the mainly pom of your project.
Archetype’s pom, that goes on:
- archetype’s root dir
This one above is self explanatory.
Creating custom Archetype
I will list here three ways that you can use to create the archetype.
From zero
The first way is from zero, where you’ll need to
- Create the archetype following the structure mentioned above.
- Install the project with
mvn install
- Generate your archetype with the command bellow
mvn archetype:generate
-DarchetypeGroupId=<archetype-groupId>
-DarchetypeArtifactId=<archetype-artifactId>
-DarchetypeVersion=<archetype-version>
-DgroupId=<project-groupId>
-DartifactId=<project-artifactId>
From some Archetype
Following this way, you’ll need to
- Generate a structure of archetype from one that already exists, like this
mvn archetype:generate
-DarchetypeGroupId=org.apache.maven.archetypes
-DarchetypeArtifactId=maven-archetype-archetype
-DgroupId=<project-groupId>
-DartifactId=<project-artifactId>
Use an existing project to create the Archetype
This way is the easiest if your project is already structured with the base files, because you just need to
mvn archetype:create-from-project
- Some adjusts may be needed when the plugin can’t generalize all the things that you need.
Generated file structure
No matter how you chose to create, your structure will be similar to this one
archetype
|-- pom.xml
|-- src
|-- main
|-- resources
|-- META-INF
| |-- maven
| |-- archetype-metadata.xml
|-- archetypes-resources
|-- pom.xml
|-- src
|-- main
| |-- java
| | |-- Application.java
| | | -- some-package
| | |-- another-package
| |-- resources
| | |-- application-dev.properties
| | |-- application-qa.properties
| | |-- application-prod.properties
|-- test
| |-- java
| | |-- ApplicationTest.java
We can use dynamic names on files, like __project-name__Application.java
, or __property-file-dev__.properties
This names will be filled with the value from archetype-descriptor.requiredProperties.requiredProperty
And here goes an example of archetype-descriptor
<archetype-descriptor name="basic">
<requiredProperties>
<requiredProperty key="project-name"/>
<requiredProperty key="project-file-name-qa">
<defaultValue>application-qa</defaultValue>
<requiredProperty>
<requiredProperty key="junit-version">
<defaultValue>5.2</defaultValue>
<requiredProperty>
</requiredProperties>
<!-- Filesets is used for folder structures -->
<fileSets>
<fileSet filtered="true">
<directory>src/main/resources</directory>
<includes>
<include>*.txt</include>
<include>*.properties</include>
</includes>
<excludes>
<exclude>**/*.xml</exclude>
</excludes>
</fileSet>
<fileSet filtered="true" packaged="true">
<directory>src/main/java</directory>
</fileSet>
<fileSet filtered="true" packaged="true">
<directory>src/test/java</directory>
</fileSet>
</fileSets>
</archetype-descriptor>
Using the archetype
Now that our archetype is created, we need to install it with Maven to the local catalog so we can use it later.
mvn clean install
Now we’re ready to generate applications using this archetype. We can achieve this by running this command line
mvn archetype:generate \
-DarchetypeGroupId=<archetype-groupId> \
-DarchetypeArtifactId=<archetype-artifactId> \
-DarchetypeVersion=<archetype-version> \
-DgroupId=<my.groupid> \
-DartifactId=<my-artifactId>
This command may differ based on which required properties you configured.
Or we can just go to the IDE and create a new project from an archetype (See https://austinsdev.com/article/2018/create-maven-project-archetype-intellij-idea)