Git Commands Guideline
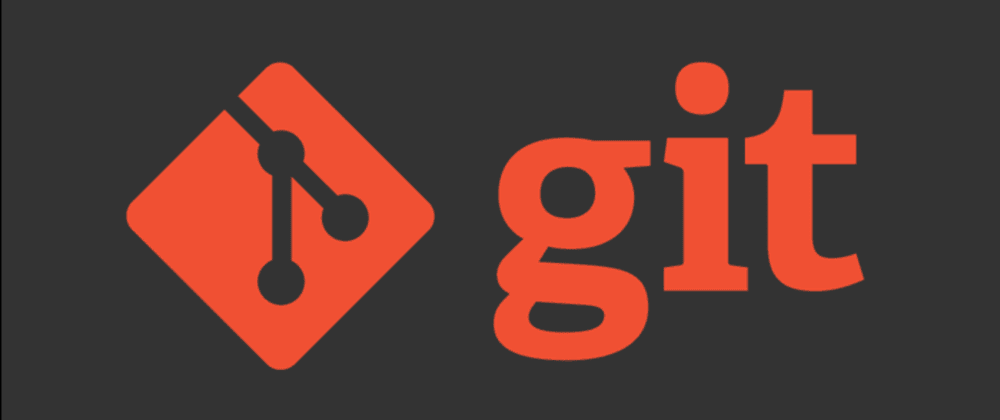
Very common git commands and some use cases where it might be useful.
This article shows some useful git commands, and when to use them.
Assumptions
It’s necessary to have git installed, and for more information about how to install git, please see this official documentation.
Scenarios
So let’s start stressing some scenarios that you might come across.
Making good commits
Commit is probably one of the most used commands of git’s cli. And making good commits helps when you need to find stuff in your repository. Well, good is something relative from person to person, but in general, a commit that is atomic, with nothing more than described on message, is a good one.
What if I add more files than needed (using git add), figured it out, and now wants to remove it?
git reset <your_unwanted_file>
Or you can remove all files from staging, using:
git reset HEAD -- .
If you want to reset some already made commit, the command is quite like these shown above, but with some differences. See the section “Resetting and reverting commits”.
Analysing commits
You may want to analyse the commits to troubleshoot something.
If you want to analyse just one file, and see all the changes on that file, and each commit that made that change, you can use:
git log --follow -p -- <your_file>
Saving work for later
Let’s suppose that you need to save your changes to work another time, removing them from your current git directory. With git, you can transfer this work to a stack and get it another time. I will call this stack as Stash, because the command to manipulate it is git stash
. You also have a bunch of commands to manipulate the Stash. Let’s see more in the next sections.
For easy understand, the verb to stash is like sweep the dust under your carpet 😆. Because you’re not getting rid of it, you will see the dust there when you remove the carpet.
Add work to Stash
First, you’ll add your current changes to the stack. You can achieve this by running
git stash
Now, your changes are saved and, in the same time, removed from your git directory. But this happened only for those files previously added to git by git add
command. If you also want to stash the files untracked by git, you can run
git stash -u
Now your untracked files was saved as well, but in another stash. Every time you run git stash, it creates another stash.
It’s also possible to stash all of your files, even the ignored ones, by running
git stash -a
List work on Stash
There is a list of stashed files, that can be viewed by running:
git stash list
We can save more friendly messages to these stashes, this command below saves a message to the last stash on the stack
git stash save "my message"
To resume the stash, we can execute
git stash show
This shows us only a brief of the stash, but if we need further information, we can run
git stash show -p
Get work from Stash
Now, what if we want these stashes back? Well, you can get the changes back to your git directory, without removing them from the stash stack, by running
git stash apply
And if you want to apply them but also remove them from stack, you can run
git stash pop
If you know anything about data structures, you know how stack works. But if you don’t, stack is LIFO (Last In, First Out). In another words, the last item that come to your stack is the first that goes out, like a stack of books, where you put the books 1, 2, 3, 4, 5, and when you pick a book, you pick the 5 first, because is the book on top of the others.
The stash is a stack, and by default when you apply/pop something from this stack, you get the first item from stash. If you want to pick an item different from the first one, you can use the following command
git stash pop stash@{1}
In this example, we are picking the second item from the stack. The first is the @{0}.
Delete from Stash
If we accidentally create a stash, and need to remove it, we can delete the stash by executing
git stash drop stash@{0}
If you need to delete all the stash, run
git stash clear
But, BE CAREFUL cleaning all your stash, since…
With great power comes great responsibility.
— Stan Lee (@TheRealStanLee) May 24, 2018
Excelsior!
Changing commits/commit history
Sometimes you may need to change a commit, like adding more files to the commit that you’ve just done, or maybe delete a commit because you made something wrong, commited on the wrong branch, or whatever the motive. There are some git commands that allows you to change your commits, and these commands change your git history. Before dive into this commands, let’s undertant a little bit more about the git commits.
Each commit has a hash, that is a unique code that identifies the commit, and in the git history, each commit points to another commit. For instance:
These commits are in chronological order, from hash1 (former) to hash5 (latter). If you execute some command that changes the commit history of the hash5, now the hashes will be like this
Please note that the hash4 point to hash6 now, that is a new hash. We changed the commit with id hash5 and this commit doesn’t exist anymore. All the commits will follow the hash6 commit, and if someone has commit hash5 on their machine, and try to commit new stuff from it, when this person tries to merge, the git will loose some stuff because there is no connection anymore between these commits.
Now let’s suppose that you already pushed that first sequence of hashes to your origin, and made some change locally that affects the history of the commits. When you try to push the new history to your origin, you’ll be blocked because the origin was expecting a new commit, like the hash5 pointing to hash6… but instead of adding a new commit, you changed the existing ones, and now the hash4 doesn’t point to hash5 anymore.
The remote repository was expecting a new hash in the link, and not a change on the linked structure of existing ones.
In these cases, a forced push is needed to achieve what you want. But remember to use it carefully. Also some of these commands that rewrites the git history are not recommended to do on public branches. The motive is because some people may create a commit, and you will erase this commit because you are changing the history and forcing git to accept the history from your machine.
Now, let’s go to code.
Saying once again, for almost all of these commands, you can execute them if you pushed your changes and also if you doesn’t had pushed yet. But if you had already pushed, a forced push (git push --force
) will be needed.
git push --force
can be irreversibleAmending commits
If you just made a commit, and you forgot to add some change, and now you want the same commit that you just made but with one more change, you can amend this commit, by running
git commit --amend
Note that you don’t need a message to this commit. This is because you will use the last commit, and the new changes will be appended to this last commit. Under the table, your commit is not edited at all. Instead, what really happens is, a new commit is created, and because of this, your last commit’s hash will change. So, if you already had pushed to remote, a push force will be needed.
Resetting and reverting commits
Roughly speaking, if you want to undo some changes already commited from your repository, you can do that in two ways, using reset or using revert. Reset will drop the commits, like they have never been made to your repository, and revert will create a new commit that undo all the changes that you did.
Resetting
Starting with reset, if you want your current git directory to go back some commits, you can use three reset strategies. Git has soft, hard, and mixed reset.
- With soft strategy: Git will go back to the commit that you specify, but keeping all the changes, and keeping them as staged.
- With mixed strategy: Git will go back to the commit that you specify, but keeping all the changes, and keeping them as unstaged. Mixed is the default strategy, executed even if no strategy is passed.
- With hard strategy: Git will go back to the commit that you specify, and will delete all your changes that came after the specified commit.
To go back just one commit, with soft strategy, use this command
git reset --soft HEAD~1
If you want to go back more than just the last commit, you can also specify the hash of the commit that you want to go back to
git reset
git reset --soft <commit_hash>
To use another strategy, just change the --soft
to --mixed
or to --hard
, according to your needs.
Reverting
Now, using the revert strategy, we won’t go back to previous commit, but instead we will create a change that goes on top of all of your commits, reverting the commits that you specify.
For instance, if you want to reset just one commit, you can use this command
git revert HEAD~1..
Or, if you want to reset the last three commits, use this
git revert HEAD~3..
As you can see, the command above tell git to revert the commits starting from HEAD, and revert three commits from there. The two dots creates a range, otherwise just the third commit, counting from the HEAD, will be reverted. The command above is equivalent
git revert HEAD~3..HEAD
You can also specify the commit hash or hashes to the commit or commits, instead of using a full range of commits.